Add OCR to your WEB pages - JavaScript - Electron
OCR on your WEB Site
More showcases >var input = Module.WasmAsposeOCRInput();
var inputs = new Module.WasmAsposeOCRInputs();
// Prepare settings
var settings = Module.WasmAsposeOCRRecognitionSettings();
input.url = "<file name>";
inputs.push_back(input);
var result = Module.AsposeOCRRecognize(inputs, settings);
// Serrialize result
var result_str = Module.AsposeOCRSerializeResult(
result, Module.ExportFormat.text);
console.log(result_str);
Why Choose Aspose.OCR for JavaScript via C++?
Aspose.OCR for JavaScript via C++ allows you to extract text from scanned pages, photos, screenshots, and other images directly on a web page or from cross-platform Electron apps. It is based on WebAssembly (Wasm) technology, which allows the code to run on the end user’s device without the need for a web server. It can be natively integrated into your JavaScript context, including access to all web browser functions. Aspose.OCR for JavaScript via C++ provides the highest security when embedded on the web, and will enforce the browser’s same-origin and permissions security policies.
Our powerful and feature rich Optical Character Recognition (OCR) API supports 28 languages based on Latin, Cyrillic and Asian scripts, including Chinese and Hindi, and can recognize files in the most popular formats. Various processing filters allow you to recognize skewed, distorted and noisy images. The recognition results are returned in the most popular data exchange formats.
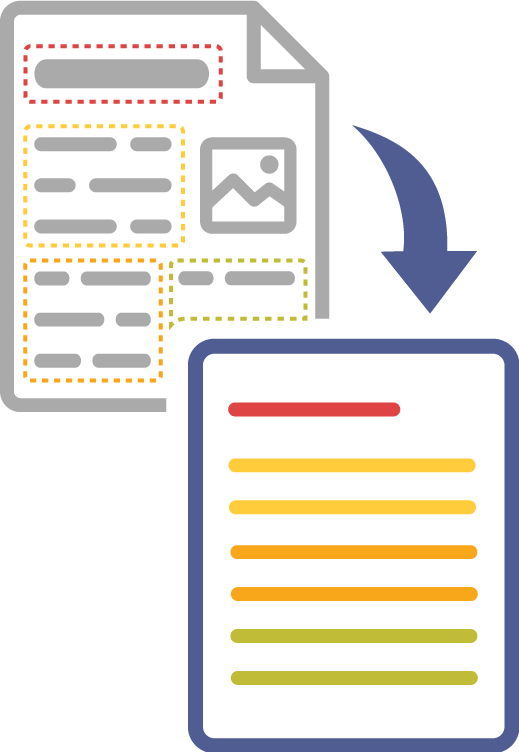
Fast and Precise OCR
Achieve high-speed and accurate OCR results with advanced JavaScript via C++ technology.
Multilingual Support
Recognize text in 28 languages, including Latin, Cyrillic, and Chinese scripts, ensuring versatility for your JavaScript applications via C++ integration.
Versatile Image Support
Process images from scanners, cameras, and smartphones effortlessly with JavaScript via C++.
Precision in Chinese Character Recognition
Recognize over 6,000 Chinese characters with precision in your JavaScript projects via C++.
Layout detection
Identify and categorize content blocks in images to ensure the correct order of extracted text, regardless of layout.
Live code sample
Initiate text recognition from images in just three lines of code. Experience the simplicity!
* By uploading your files or using the service you agree with our Terms of use and Privacy Policy.
Convert image to text
More examples >const fileData = new Uint8Array(e.target.result);
let filename = file.name;
let stream = Module.FS.open(filename, "w+");
Module.FS.write(stream, fileData, 0, fileData.length, 0);
Module.FS.close(stream);
var input = Module.WasmAsposeOCRInput();
var inputs = new Module.WasmAsposeOCRInputs();
var settings = Module.WasmAsposeOCRRecognitionSettings();
input.url = filename;
inputs.push_back(input);
var result = Module.AsposeOCRRecognize(inputs, settings);
// Get recognition results as text
var result_str = Module.AsposeOCRSerializeResult(result, Module.ExportFormat.text);
console.log(result_str);
OCR Integration in your WEB application
Aspose.OCR for JavaScript allows the code to run directly in the end user’s web browser (client-side) or in browser-based environments, such as Electon.
Supported file formats
Aspose.OCR for Javascript via C++ can work with virtually any file you can get from a scanner or camera. Recognition results are returned in the most popular file and data exchange formats that can be saved, imported to a database, or analyzed in real time.
Images
- JPEG
- PNG
- TIFF
- BMP
Batch OCR
- ZIP
Recognition results
- Text
- JSON
- XML
Effortless Installation
Aspose.OCR for JavaScript via C++ is distributed as a self-contained downloadable package which does not require any external dependencies to be installed. Just unpack it next to your HTML page, and you’re ready to convert any image to text right in the browser.
Request a trial license to kickstart the development of a fully functional OCR application without limitations.
28 Recognition Languages
JavaScript OCR API recognizes 28 languages and popular writing scripts, including mixed languages:
Leave language detection to the library or define the language yourself for enhanced recognition performance and reliability.
- Extended Latin alphabet: Croatian, Czech, Danish, Dutch, English, Estonian, Finnish, French, German, Italian, Latvian, Lithuanian, Norwegian, Polish, Portuguese, Romanian, Slovak, Slovenian, Spanish, Swedish;
- Cyrillic alphabet: Belorussian, Bulgarian, Kazakh, Russian, Serbian, Ukrainian;
- Chinese: Over 6,000 characters;
- Hindi.
Suitable for any content
JavaScript OCR API recognizes 28 languages and popular writing scripts, including mixed languages:
Leave language detection to the library or define the language yourself for enhanced recognition performance and reliability.
Key Features
Aspose.OCR for Javascript via C++ Explore the advanced features of Aspose.OCR for JavaScript.
Photo OCR
Extract text from smartphone photos with scan-level accuracy.
Searchable PDF
Convert any scan into a fully searchable and indexable document.
URL recognition
Recognize an image from URL without downloading it locally.
Bulk recognition
Read all images from multi-page documents, folders and archives.
Any font and style
Identify and recognize text in all popular typefaces and styles.
Fine-tune recognition
Adjust every OCR parameter for best recognition results.
JavaScript Code Samples
Discover code samples to seamlessly integrate Aspose.OCR for JavaScript via C++ into your HTML pages and Electron apps.
Simple Installation for JavaScript
Image Recognition with JavaScript
The widespread adoption of OCR applications is usually stopped by the fact that scanners are not commonplace for most users. Our OCR library has powerful built-in image pre-processing filters that can handle dark, rotated, skewed, and noisy images. In combination with support for all image formats, it allows for reliable recognition of even smartphone photos. Most of the pre-processing and image correction is done automatically, so you will only have to intervene in difficult cases.
Apply automatic image corrections - JavaScript
// Load photo from user input
const fileData = new Uint8Array(e.target.result);
let filename = file.name;
let stream = Module.FS.open(filename, "w+");
Module.FS.write(stream, fileData, 0, fileData.length, 0);
Module.FS.close(stream);
var input = Module.WasmAsposeOCRInput();
input.url = filename;
// Automatically adjust contrast and remove noise
var settings = Module.WasmAsposeOCRRecognitionSettings();
settings.detect_areas_mode = Module.DetectAreasMode.PHOTO;
settings.auto_contrast = true;
settings.auto_denoising = true;
// Extract text from photo:
var inputs = new Module.WasmAsposeOCRInputs();
inputs.push_back(input);
var result = Module.AsposeOCRRecognize(inputs, settings);
// Output recognition results
var result_str = Module.AsposeOCRSerializeResult(result, Module.ExportFormat.text);
console.log(result_str);
Universal image to text Converter for JavaScript
While many businesses, organizations and individuals have been actively working on reducing their reliance on paper documents, this is still the most widespread format for storage and sharing. Scanned documents backed by physical archives are sufficient for regulatory compliance, legal purposes, long-term backup and redundancy. However, business cases frequently arise for creating new content based on existing scanned content or portions of existing documents. Aspose.OCR for JavaScript via С++ makes it easy to convert a scanned page into a searchable and editable text. Content is recognized with high accuracy and speed, saving you the time and effort of manual typing and ensuring there are no human errors, especially when working with large volumes of information.
Convert image to document - JavaScript
// Load a scanned page from user input
const fileData = new Uint8Array(e.target.result);
let filename = file.name;
let stream = Module.FS.open(filename, "w+");
Module.FS.write(stream, fileData, 0, fileData.length, 0);
Module.FS.close(stream);
var input = Module.WasmAsposeOCRInput();
input.url = filename;
// Analyze content structure
var settings = Module.WasmAsposeOCRRecognitionSettings();
settings.detect_areas_mode = Module.DetectAreasMode.DOCUMENT;
settings.upscale_small_font = true;
// Extract text from a page
var inputs = new Module.WasmAsposeOCRInputs();
inputs.push_back(input);
var result = Module.AsposeOCRRecognize(inputs, settings);
var editableText = Module.AsposeOCRSerializeResult(result, Module.ExportFormat.text);
Extracting numeric data from tables
When managing large printed tables containing numerical data, such as raw data of field sociological surveys or inventory lists, manual extraction becomes a sluggish, impractical process highly susceptible to human errors. OCR helps to automate and standardize the extraction of information, ensuring consistent and reliable results. Aspose.OCR for JavaScript via С++ fully automates conversion of scanned or photographed tabular data into machine-readable content. Extracted data can be easily integrated into databases and analyzed, contributing to more informed decision-making.
Table image to text - JavaScript
// Load a scan or photo from user input
const fileData = new Uint8Array(e.target.result);
let filename = file.name;
let stream = Module.FS.open(filename, "w+");
Module.FS.write(stream, fileData, 0, fileData.length, 0);
Module.FS.close(stream);
var input = Module.WasmAsposeOCRInput();
input.url = filename;
// Analyze tabular structures
var settings = Module.WasmAsposeOCRRecognitionSettings();
settings.detect_areas_mode = Module.DetectAreasMode.TABLE;
// Limit the subset of characters to improve recognition accuracy and increase performance
settings.alphabet = "1234567890.,;";
// Extract text from a table
var inputs = new Module.WasmAsposeOCRInputs();
inputs.push_back(input);
var result = Module.AsposeOCRRecognize(inputs, settings);
var editableText = Module.AsposeOCRSerializeResult(result, Module.ExportFormat.text);