Convert OneNote to Notion via C#
Export Microsoft® OneNote to Notion on .NET Framework, .NET Core.
How to Convert OneNote to Notion Using C#
In order to convert OneNote to Notion, we’ll use Aspose.Note for .NET API which is a feature-rich, powerful and easy to use document manipulation and conversion API for C# platform. Open NuGet package manager, search for Aspose.Note and install. You may also use the following command from the Package Manager Console.
Package Manager Console Command
PM> Install-Package Aspose.Note
Steps to Export Text Data from OneNote to Notion
In this tutorial, we will guide you through the process of transferring text data from OneNote to Notion using C#. We will cover four main steps: retrieving text data from a OneNote document, connecting to your Notion account, creating a new page in Notion, and finally combining all these steps into a single script for transferring text data, you will need the following libraries Aspose.Note and Notion.Net
Steps to Export Text Data from OneNote to Notion
Step 1: Retrieving Text Data from OneNote
Below is an example code snippet to extract all text data from a OneNote document:
var documentPath = "";
var document = new Document(documentPath);
foreach (var oneNotePage in document)
{
var oneNoteAllRichText = oneNotePage.GetChildNodes<RichText>();
}
Step 2: Connecting to Your Notion Account
Before getting started, you need to create an integration and find the token. You can learn more about authorization here .
var authToken = "";
var client = NotionClientFactory.Create(new ClientOptions
{
AuthToken = authToken
});
Step 3: Obtaining the Parent Page Identifier in Notion
Click on the three dots on the parent page in Notion and get the link to the page. The link will contain the identifier, which you will need for the next step.
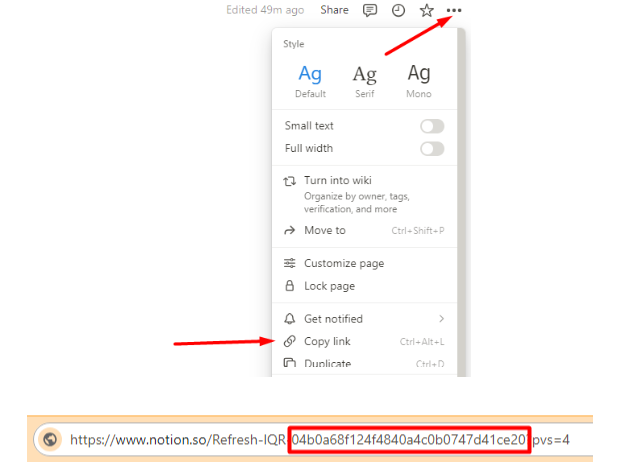
Step 4: Creating a New Page in Notion
The code below is intended for creating a new page in Notion:
var authToken = "";
var parentPageId = "";
var client = NotionClientFactory.Create(new ClientOptions
{
AuthToken = authToken
});
var page = await client.Pages.RetrieveAsync(parentPageId);
var pagesCreateParametersBuilder = PagesCreateParametersBuilder
.Create(new ParentPageInput { PageId = page.Id })
.AddProperty("title",
new TitlePropertyValue
{
Title = new List<RichTextBase>
{
new RichTextTextInput { Text = new Text { Content = “New page title”} }
}
});
var pagesCreateParameters = pagesCreateParametersBuilder.Build();
var pageCreate = await client.Pages.CreateAsync(pagesCreateParameters);
Step 5: Combining All Steps
Combining all the previous steps, we get the following code for transferring text data from OneNote to Notion:
public static async Task Main(string[] args)
{
var documentPath = "";
var authToken = "";
var parentPageId = "";
var document = new Document(documentPath);
var client = NotionClientFactory.Create(new ClientOptions
{
AuthToken = authToken
});
var page = await client.Pages.RetrieveAsync(parentPageId);
foreach (var oneNotePage in document)
{
var oneNoteAllRichText = oneNotePage.GetChildNodes<RichText>();
var pagesCreateParametersBuilder = PagesCreateParametersBuilder
.Create(new ParentPageInput { PageId = page.Id })
.AddProperty("title",
new TitlePropertyValue
{
Title = new List<RichTextBase>
{
new RichTextTextInput { Text = new Text { Content = oneNotePage.Title.TitleText.Text } }
}
});
foreach (var richText in oneNoteAllRichText)
{
if (richText.IsTitleDate || richText.IsTitleText || richText.IsTitleTime)
{
continue;
}
pagesCreateParametersBuilder.AddPageContent(new ParagraphBlock
{
Paragraph = new ParagraphBlock.Info
{
RichText = new List<RichTextBase> {
new RichTextText
{
Text = new Text { Content = richText.Text }
}
}
}
});
}
var pagesCreateParameters = pagesCreateParametersBuilder.Build();
var pageCreate = await client.Pages.CreateAsync(pagesCreateParameters);
}
}
By following these steps and utilizing the provided code snippets, you can seamlessly transfer text data from OneNote to Notion using C#.
A standalone OneNote Document Manipulation Library capable of reading, creating, editing, and converting Microsoft OneNote files. The OneNote API also allows loading documents, rendering text and images, navigating through the document object model, extracting text from any part of a file, and more.
About Microsoft OneNote files
File represented by .ONE extension are created by Microsoft OneNote application. OneNote lets you gather information using the application as if you are using your draftpad for taking notes. OneNote files can contain different elements that can be placed at non-fixed locations on document pages. These elements may contain text, digitized handwriting, and objects copied from other applications including images, drawings and multimedia (audio/video) clips. Microsoft now offers online version of OneNote as part of Office365 where Notes can be shared with other OneNote users over the internet.
About Notion file format
Unfortunately, Notion doesn’t have a single, specific file format like traditional documents. It uses a proprietary database format to store and manage your information. Notion operates as an all-in-one workspace that combines note-taking, task management, and collaboration. Also worth noting it’s important to note that Notion primarily functions as a cloud-based service, and the end-users often interact with the data through the Notion app or web interface rather than dealing with specific file formats directly. This means you can’t directly open or edit individual Notion pages in other software.
Other Supported OneNote Conversions by .NET
You can also convert OneNote to many other file formats: